[flutter]
Implement a button that moves to the top of the list in 3 minutes
3분만에 리스트 최상단으로 이동하는 버튼 구현하기 (Scroll Back To Top)
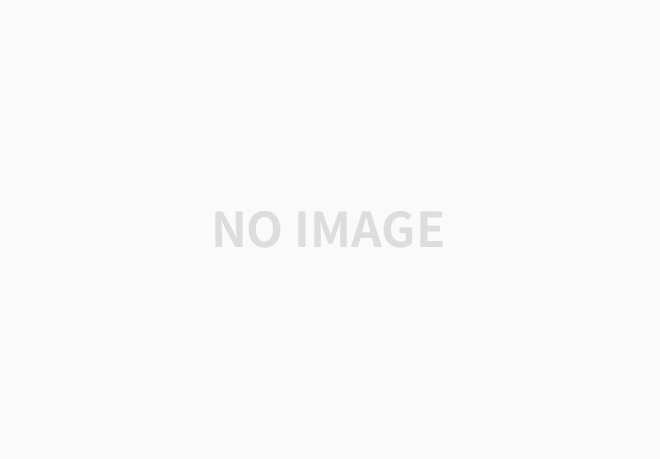
설명 (Explanation)
리스트 최상단으로 이동하는 버튼 (Scroll Back To Top)은 사용자에게 있어서 큰 편리함을 제공한다.
리스트를 탐색하다가 한 번에 리스트의 가장 위로 이동할 수 있기 때문이다.
우리는 이 버튼을 플로팅 버튼으로 구현할 것이며
리스트 스크롤이 일정한 거리만큼 내려갔을 때부터 버튼이 표시되도록 할 것이다.
(
The button that moves to the top of the list (Scroll Back To Top) provides great convenience to users.
This is because you can move to the top of the list at once while traversing the list.
We will implement this button as a floating button
We'll make the button appear when the list scrolls down a certain distance.
)
코드 (source code)
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
// 상단이동 버튼이 표시(visible)되어야하는지 판단용 변수
bool _showBackToTopButton = false;
// 스크롤 컨트롤러
late ScrollController _scrollController;
@override
void initState() {
_scrollController = ScrollController()
..addListener(() {
setState(() {
if (_scrollController.offset >= 400) {
_showBackToTopButton = true; // 버튼 표시(visible = true)
} else {
_showBackToTopButton = false; // 버튼 숨김(visible = false)
}
});
});
super.initState();
}
@override
void dispose() {
_scrollController.dispose(); // 컨트롤러 메모리 해제
super.dispose();
}
// 스크롤 최상단 이동 버튼을 눌렀을 때, 리스트의 최상단으로 이동하는 코드
void _scrollToTop() {
_scrollController.animateTo(0,
duration: const Duration(seconds: 3), curve: Curves.linear);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: SingleChildScrollView(
controller: _scrollController,
child: Column(
children: [
// 스크롤 최상단 이동 테스트를 위한 더미 리스트
Container(
height: 600,
color: Colors.amber,
),
Container(
height: 600,
color: Colors.blue[100],
),
Container(
height: 600,
color: Colors.red[200],
),
Container(
height: 600,
color: Colors.orange,
),
Container(
height: 600,
color: Colors.yellow,
),
Container(
height: 1200,
color: Colors.lightGreen,
),
],
),
),
// 실제로 UI에 보여지는 최상단 이동 버튼 코드
floatingActionButton: _showBackToTopButton == false
? null
: FloatingActionButton(
onPressed: _scrollToTop, // 버튼을 눌렀을 때 어떤 함수를 돌릴 것인지 설정
child: const Icon(Icons.arrow_upward), // 버튼에 표시되는 아이콘 모양 설정
),
);
}
}
위 코드를 main.dart에 복사하고 플러터 프로젝트를 실행하면,
형형색색의 네모칸의 리스트와 스크롤을 내렸을 때 우리가 구현하고자 하는 버튼이 나타난다.
이 버튼을 누르면 자동으로 리스트의 최상단으로 이동하는 것을 확인할 수 있다!
(
Copy the above code to main.dart and run the flutter project,
A list of colorful squares and a button we want to implement appear when we scroll down.
If you press this button, you can see that it automatically moves to the top of the list!
)